Introduction About Video Player –
Today we are going to learn one more important part of the react native app which is “How To Implement Video Player in React Native“.
If you are here then you know about the media player.
Output result –
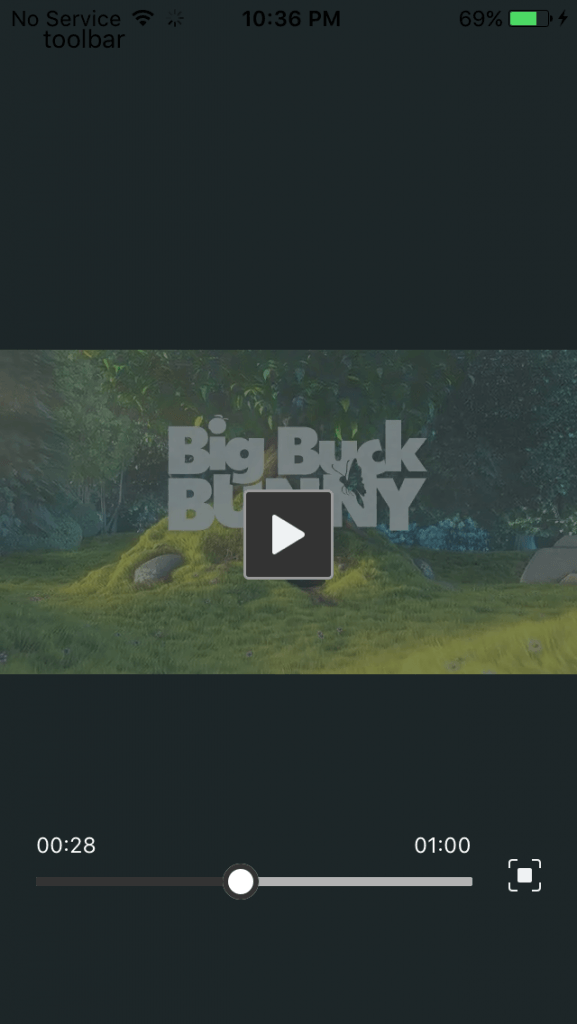
For implementing a video player we are going to use the video player library.
Many other libraries are available for showing the media in react-native but I like this one and it has a great number of GitHub stars too.
So let’s start the implementation.
Step 1 – Installation
First, we need to install the third-party video npm plugin.
npm install react-native-video
or
yarn add react-native-video
Step 2 – Import
After installing the npm plugin successfully, now we need to import that plugin into your screen.
import Video from 'react-native-video';
Step 3 – Design the render
Now we set up the view for showing the video.
<Video
//for playing local videos
// source={
// this.state.showLocal ?
// require('../basic/broadchurch.mp4') :
// {
// uri: "https://rawgit.com/mediaelement/mediaelement-files/master/big_buck_bunny.mp4"
// }
// }
source={{
uri: 'https://rawgit.com/mediaelement/mediaelement-files/master/big_buck_bunny.mp4',
}} // Can be a URL or a local file.
ref={ref => {
this.player = ref;
}} // Store reference
//for showing a static image
//poster="https://upload.wikimedia.org/wikipedia/commons/thumb/3/34/English_Cocker_Spaniel_4.jpg/800px-English_Cocker_Spaniel_4.jpg"
// audioOnly={true} //for only audio playing
controls={true}
onBuffer={this.onBuffer} // Callback when remote video is buffering
// onError={this.videoError} // Callback when video cannot be loaded
onEnd={() => {
this.player.seek(0);
}}
onError={err => {
Alert.alert(JSON.stringify(err));
}}
style={styles.backgroundVideo}
/>
Step 5 – Complete code
Here I put the complete code of mine, so you can directly copy and paste it, and you see the result. there is some code which is commented in the file, uncomment those based on your condition
import React, {Component} from 'react';
import {
View,
ScrollView,
Dimensions,
Alert,
TouchableOpacity,
StyleSheet,
} from 'react-native';
import Video from 'react-native-video';
export default class MediaPlayerScreen extends Component {
constructor(props) {
super(props);
this.state = {
is_device: false,
};
}
videoBuffer = isBuffer => {
console.log(isBuffer);
//here you could set the isBuffer value to the state and then do something with it
//such as show a loading icon
};
render() {
// const earthVideo = require('./earth.mp4');
return (
<ScrollView style={styles.container}>
<View style={styles.mainView}>
<Video
//for playing local videos
// source={
// this.state.showLocal ?
// require('../basic/broadchurch.mp4') :
// {
// uri: "https://rawgit.com/mediaelement/mediaelement-files/master/big_buck_bunny.mp4"
// }
// }
source={{
uri: 'https://rawgit.com/mediaelement/mediaelement-files/master/big_buck_bunny.mp4',
}} // Can be a URL or a local file.
ref={ref => {
this.player = ref;
}} // Store reference
//for showing a static image
//poster="https://upload.wikimedia.org/wikipedia/commons/thumb/3/34/English_Cocker_Spaniel_4.jpg/800px-English_Cocker_Spaniel_4.jpg"
// audioOnly={true} //for only audio playing
controls={true}
onBuffer={this.onBuffer} // Callback when remote video is buffering
// onError={this.videoError} // Callback when video cannot be loaded
onEnd={() => {
this.player.seek(0);
}}
onError={err => {
Alert.alert(JSON.stringify(err));
}}
style={styles.backgroundVideo}
/>
<View style={styles.absoluteOverlay}>
<TouchableOpacity
onPress={async () => {
let response = await this.player.save();
let uri = response.uri;
console.warn('Download URI', uri);
}}
text="Save"
/>
<TouchableOpacity
onPress={() => {
this.setState(state => ({showLocal: !state.showLocal}));
}}
text={this.state.showLocal ? 'Show Remote' : 'Show Local'}
/>
</View>
</View>
</ScrollView>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 10,
backgroundColor: colors.lightBlack,
// alignContent: 'center',
// justifyContent: 'center',
},
mainView: {
flex: 1,
// height: moderateScale(300),
width: '100%',
},
});
Step 6 – Methods
Following are the methods provided by the video player library.
This video player provides all the above props which you need while playing a video and we can control them easily.
Step 6 – Props
Following are the props provided by the video player library.
To check all the props please visit – https://www.npmjs.com/package/react-native-video
Step 7 – Run the project
Now you can run your project on android or ios and check your view.
react-native run-android
react-native run-ios
So we completed the react-native video playing functionality which is “How To Implement Video Player in React Native“
You can find my next post here.
You can find my post on medium as well click here please follow me on medium as well.
If have any query or issues, please feel free to ask.
Happy Coding Guys.
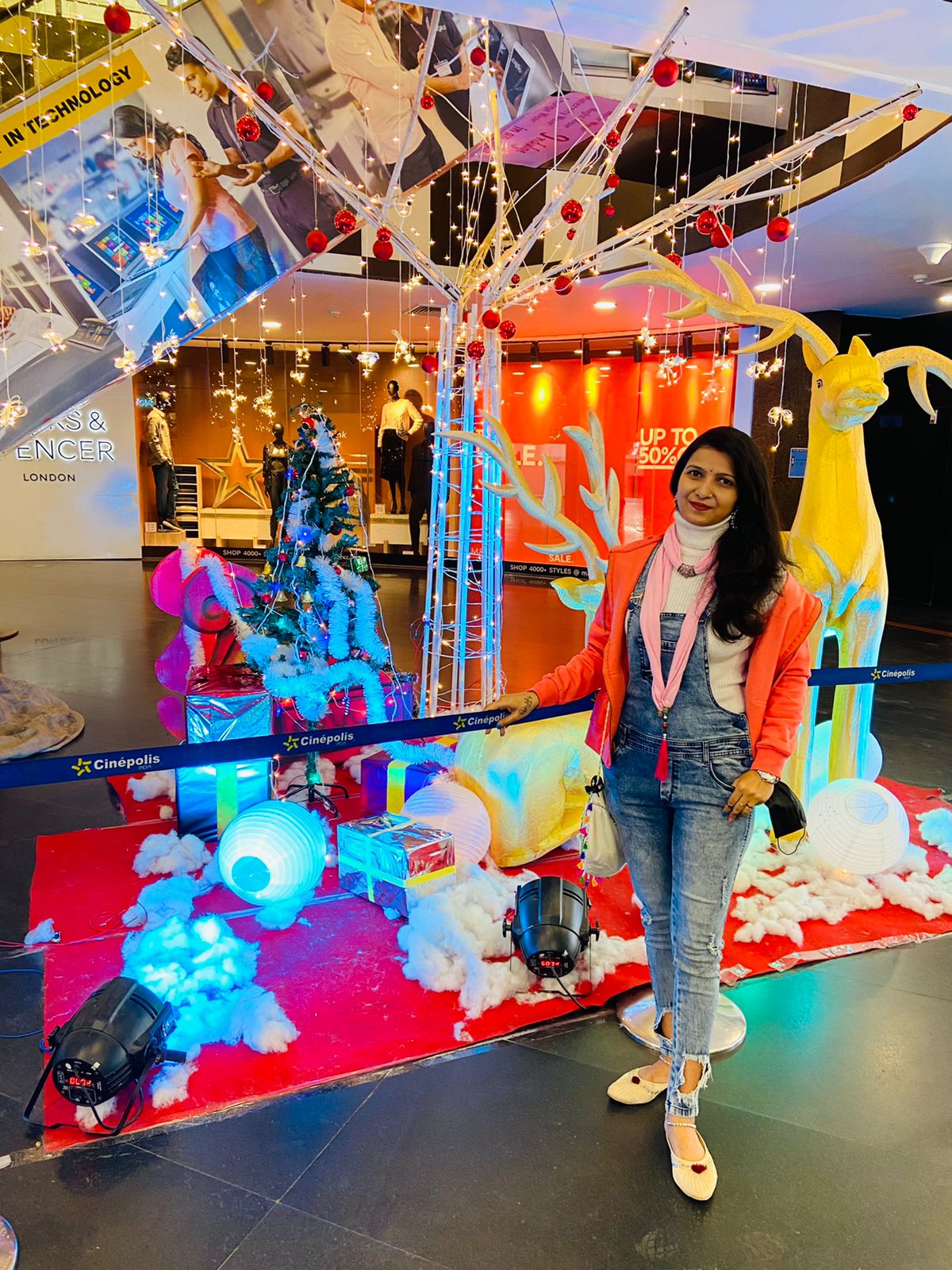