Introduction –
Today we are going to discuss about “How to Download Pdf File in React Native“, downloading is a very important part of any app, transfer data directly from/to storage without BASE64 bridging.
After completing this tutorial you able to download any image, pdf and any other file, you need only url of that file. and you can save it in your device also it shows the download indication in bar.
Output Example –
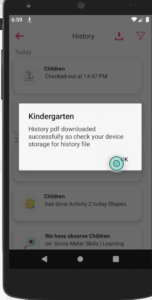
I’m a big fan of React Native, because it is very easy to implement complicated concepts and designs, in below example i am going to explain you in detail how to create a very simple image downloading functionality.
Follow all the steps carefully:
Step 1 – Plugin Installation
For downloading any image or pdf we are going to use the npm plugin rn-fetch-blob .
npm i rn-fetch-blob
Step 2 – Import The Plugin
You can import the plugin where you want to integrate the download functionality.
import RNFetchBlob from 'rn-fetch-blob';
Step 3 – View Design
Now we are going to design the view by using which we perform the download operation.
render(){
return(
<TouchableOpacity onPress={() => {
this.historyDownload();
}}
style={{
height:(50),
shadowOffset: {width: 0, height: 0},
shadowOpacity: 1,
shadowRadius:(8),
elevation:(8),
borderRadius:(25),
alignItems: 'center',
justifyContent: 'space-between',
flexDirection: 'row',
paddingLeft:(20),
paddingRight:(20),
}}>
<Text
style={{
color: Colors.titleFont,
fontSize:(16),
}}>
Download Data
</Text>
</TouchableOpacity>
)
}
Step 4 – Permission Checking
First you need to asking about download permission and also check the platform.
historyDownload() {
//Function to check the platform
//If iOS the start downloading
//If Android then ask for runtime permission
if (Platform.OS === 'ios') {
this.downloadHistory();
} else {
try {
PermissionsAndroid.request(
PermissionsAndroid.PERMISSIONS.WRITE_EXTERNAL_STORAGE,
{
title:'storage title',
message:'storage_permission',
},
).then(granted => {
if (granted === PermissionsAndroid.RESULTS.GRANTED) {
//Once user grant the permission start downloading
console.log('Storage Permission Granted.');
this.downloadHistory();
} else {
//If permission denied then show alert 'Storage Permission
Not Granted'
Alert.alert('storage_permission');
}
});
} catch (err) {
//To handle permission related issue
console.log('error', err);
}
}
}
Step 5 – Definition of function
Here we give the definition of download function, for downloading any file or image.
async downloadHistory() {
const { config, fs } = RNFetchBlob;
let PictureDir = fs.dirs.PictureDir;
let date = new Date();
let options = {
fileCache: true,
addAndroidDownloads: {
//Related to the Android only
useDownloadManager: true,
notification: true,
path:
PictureDir +
'/Report_Download' +
Math.floor(date.getTime() + date.getSeconds() / 2),
description: 'Risk Report Download',
},
};
config(options)
.fetch('GET', url)
.then((res) => {
//Showing alert after successful downloading
console.log('res -> ', JSON.stringify(res));
alert('Report Downloaded Successfully.');
});
}
Step 6 – Android Permission
You need to take a permission from user to access his device space, so go to the YourProject -> android -> app -> main -> AndroidMnifest.xml
<uses-permission
android:name="android.permission.WRITE_EXTERNAL_STORAGE"
/>
So we completed the react-native download functionality which is “How to Download Pdf File in React Native“.
You can find my post on medium as well click here please follow me on medium as well.
You can find my next post here.
If have any query/issue, please feel free to ask.
Happy Coding Guys.
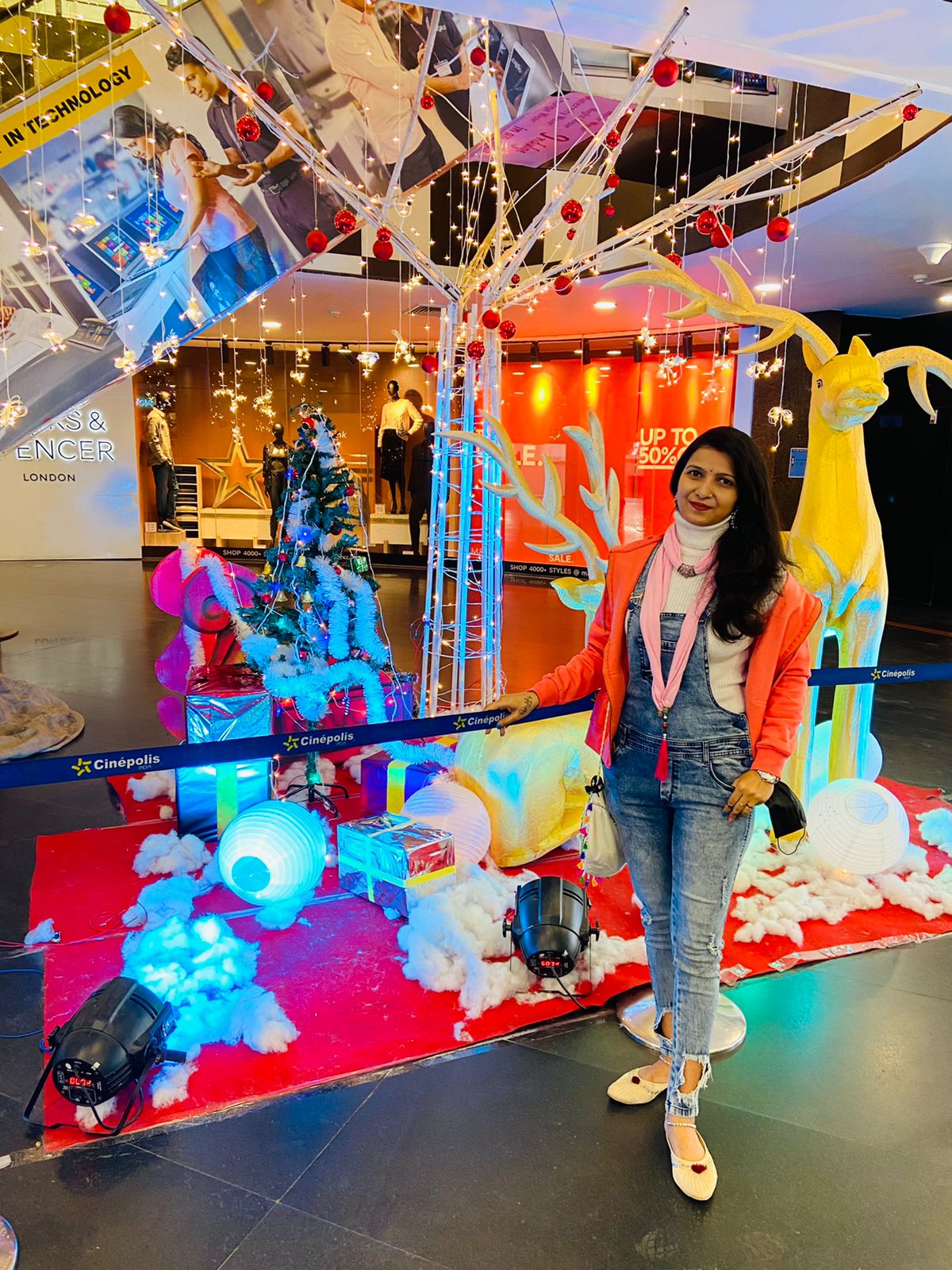