What is Crashlytics –
Here we are going to discuss the “How to integrate firebase crashlytics in react native with android app“.
Crashlytics is a lightweight, realtime crash reporting tool that helps you to track, prioritise, and fix stability issues, which improve your app quality.
Crashlytics saves your troubleshooting time by intelligently grouping your app crashes and highlighting the circumstances of crashing.
Example Output –
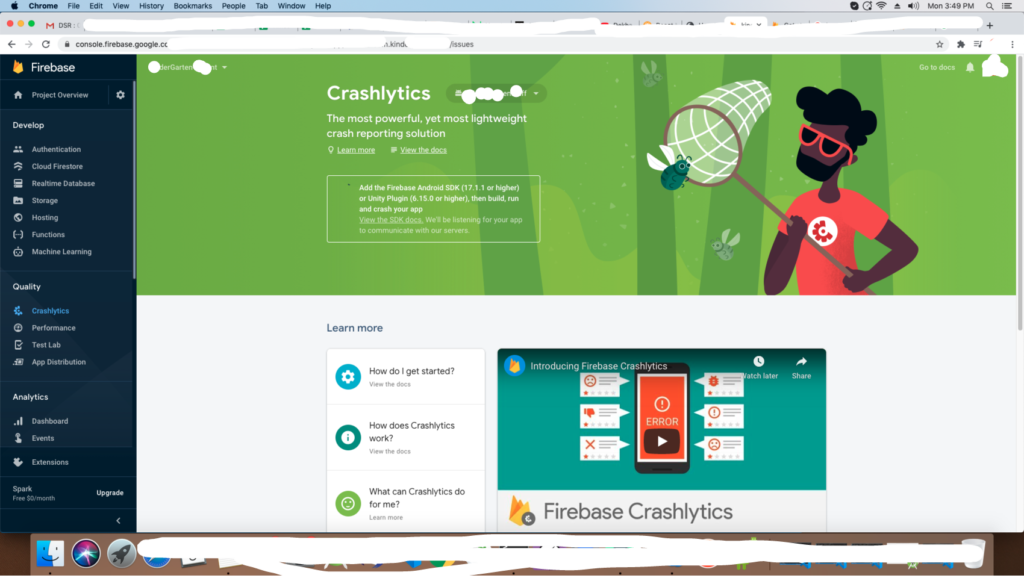
Key capabilities –
Curated crash reports | Crashlytics listed the app crashes into a manageable list of issues, provides the information and highlights the reason of crashes so you can pinpoint the root cause faster. |
Cures for the common crash | Crashlytics offers Crash Insights, where you find the helpful tips that highlight common stability problems and provide resources that make them easier to troubleshoot. |
Integrated with Analytics | Crashlytics can capture your app’s errors as app_exception events in Analytics. The events simplify debugging by giving you access a list of other events leading up to each crash, and provide audience insights by letting you pull Analytics reports for users with crashes. |
Realtime alerts | Get realtime alerts for new issues and growing issues that might require immediate attention. |
Implementation path
Connect your app | Start by adding Firebase to your app in the Firebase console. | |
Integrate the SDK | Add the Crashlytics SDK via CocoaPods or Gradle, and Crashlytics starts collecting reports. | |
Check reports in the Firebase console | Visit the Firebase console to track, prioritize, and fix issues in your app. |
Discussion part is over here, now you have much knowledge about crashlytics, so let’s start the integration of crashlytics, we are going to follow the below steps.
Step 1: Register your app with Firebase
- Open the Firebase console.
- Add your project.
- Enter your project name and provide the app package name.
- Follow the all other steps in the Firebase console, then click Create project.
- Download google-services.json
- Move the google-services.json file into the
app
directory of your project.
And for more detail please –
Enabling firebase crashlytics in your project –
For enable you’r firebase crashlytics follow these steps.
- Click on side-menu Quality -> Crashlytic
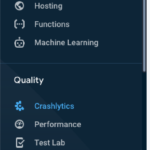
- Here you see the all you’r app and a dropdown menu, if you have more than one app then please select the app in which you want to add the crashlytics and click on the button enable, after that you see the loader. this loader is shows till that you’r app is successfully implement the crashlytics.
it look like –
Step 2: Add the Firebase plugin in Android Studio
1) Adding the crashlytics and fabric in build.gradle(project level)
- Open project – level
build.gradle
(project_location/android/build.gradle) file and add below classpaths in dependencies
dependencies {
classpath('com.android.tools.build:gradle:3.5.2')
classpath 'com.google.gms:google-services:4.2.0'
//add these 2 lines here
classpath 'com.google.firebase:firebase-crashlytics-
gradle:2.2.0'
classpath 'io.fabric.tools:gradle:1.+'
}
Note – Make sure you have added google()
in build script repositories and all project repositories.
2) Adding the maven in build.gradle file(project level) –
- And you need to add the fabric in your project for maven, so add the fabric in your repositories within build script
- URL is –
maven {
url 'https://maven.fabric.io/public'
}
- After adding all these your build.gradle buildscript is something look like –
buildscript {
ext {
buildToolsVersion = '28.0.3'
minSdkVersion = 16
compileSdkVersion = 28
/* groovylint-disable-next-line DuplicateNumberLiteral */
targetSdkVersion = 28
}
repositories {
google()// check for this line
jcenter()
//add these lines for fabric
maven {
url 'https://maven.fabric.io/public'
}
}
dependencies {
classpath('com.android.tools.build:gradle:3.5.2')
classpath 'com.google.gms:google-services:4.2.0'
classpath 'com.google.firebase:firebase-crashlytics-
gradle:2.2.0'
classpath 'io.fabric.tools:gradle:1.+'
}
}
3) Adding the Fabric in build.gradle file(App level) –
- Open app level
build.gradle
file apply fabric (project-location/android/app/build.gradle)
apply plugin: 'com.android.application'
//add this line
apply plugin: 'io.fabric'
- Add the Crashlytics dependencies in the same file(means
build.gradle
)
// Recommended: Add the Firebase SDK for Google Analytics.
implementation 'com.google.firebase:firebase-analytics:17.5.0'
// Add the Firebase Crashlytics SDK.
implementation 'com.google.firebase:firebase-crashlytics:17.2.2'
Step 3: Add the fabric plugin in react native app
Now we are going to add the fabric plugin in our react native project.
1) Plugin installation –
npm install react-native-fabric
npm i @react-native-firebase/crashlytics
npm i @react-native-firebase/app
2) Import the Plugin –
We are going to import the plugin in App.js file and force the app for crash, so we can check the firebase.
import crashlytics from '@react-native-firebase/crashlytics';
3) Force for crash –
Put the following code at the start of your app.js so we force our app for crashing.
crashlytics().crash()
Step 4: Checking the firebase for crash report
So here you need to check the firebase for see the crashing, if crashlytics successfully added in your app then the loader stop the loading and screen is look like –
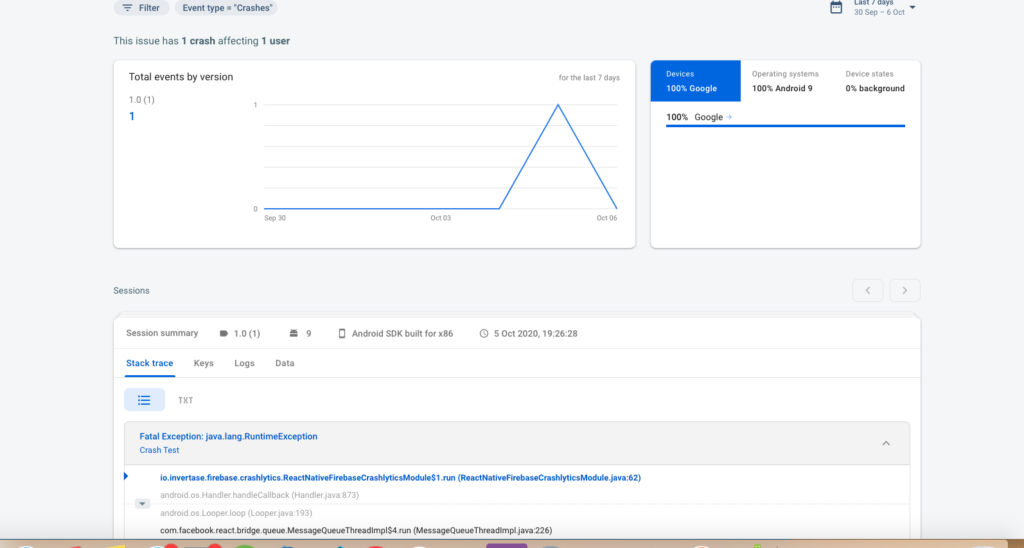
Expected Issue –
Could not find io.fabric.tools:gradle:1.31.2.
Solution –
Open the build. gradle(project level) and check for the –
maven { url ‘https://maven.fabric.io/public’ }
buildscript {
repositories {
maven { url 'https://maven.fabric.io/public' }
}
dependencies {
classpath 'io.fabric.tools:gradle:1.+'
}
}
apply plugin: 'com.android.application'
apply plugin: 'io.fabric'
So we completed the react native crashlytics setup in this tutorial which is “How to integrate firebase crashlytics in react native with android app“.
You can find my post on medium as well click here please follow me on medium as well.
You can find my next post here.
If have any query/issue, please feel free to ask.
Happy Coding Guys.
Reference link for android setup
Prefer this – link for iOS
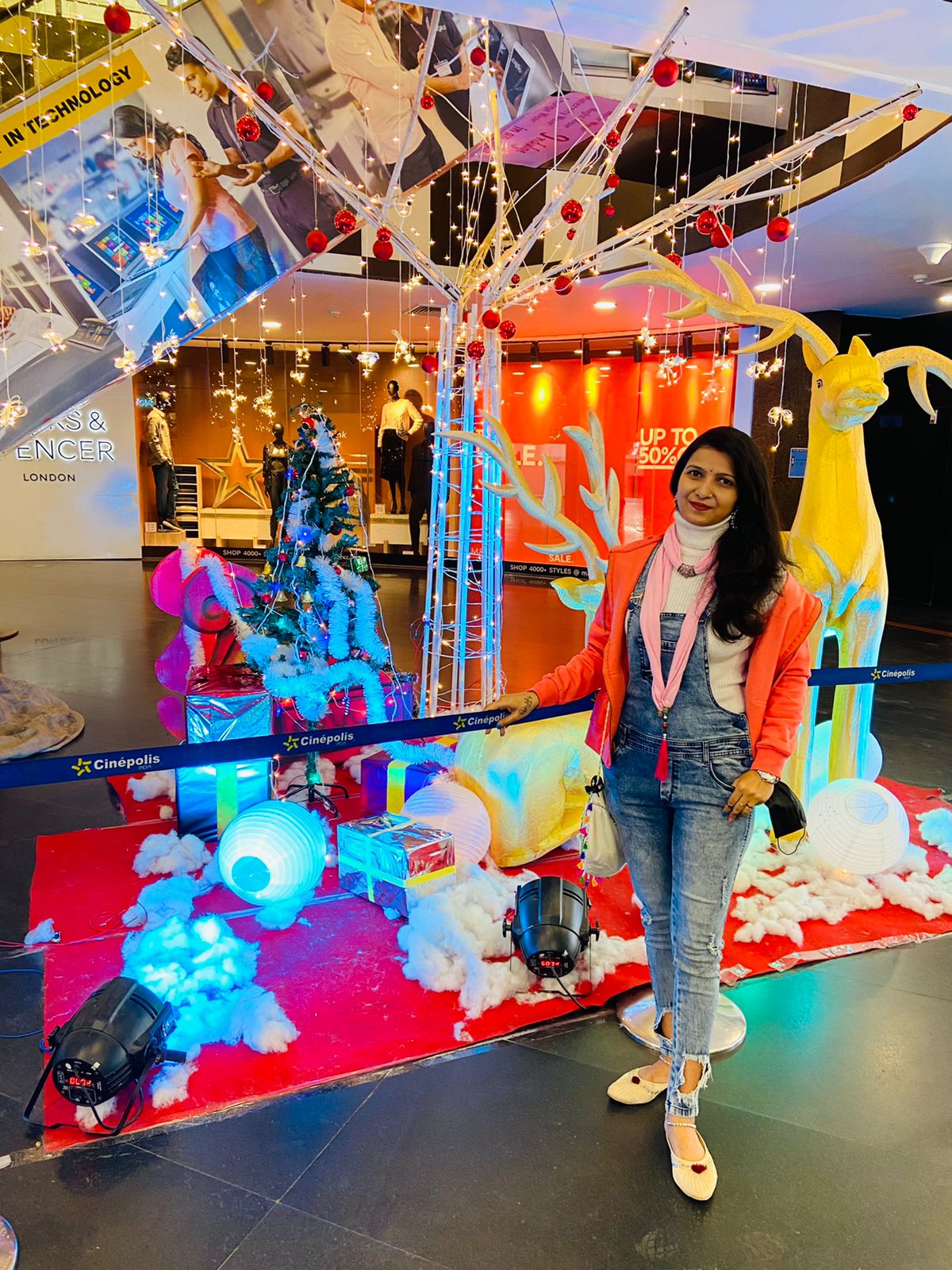