Introduction –
Here we are going to discuss about “How to implement search in react native“.
I’ll choose this topic because in internet i am not finding a single tutorial which perform the search operation without using any third party library.
Example output –
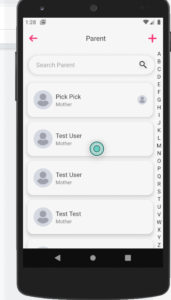
Logic behind this
- We will load the list from the api call or you can make a custom array and then show it to the user.
- User can search the data by entering the text in search bar.
- After inserting the text we perform search on each text.
- We compare the inserted data with list of data.
- Then we will make store this data into state.
- After that we will re-render the list and the user will be able to see the filtered data.
So let’s start and follow each steps carefully.
Step 1 – Declaring state
Here we declare the state for storing search data
this.state = {
parentData: [],//storing API response
isSearch: false,
isAddParent: false,
searchText: '',
};
Step 2 – Design view for search bar
Here we are going to design the search bar using textInput with search bar icon also search and cross icon showing conditionally.
render() {
return (
<View
style={{
margin: moderateScale(15),
marginRight: moderateScale(30),
width: '90%',
shadowColor: Colors.shadow,
shadowOffset: {width: 0, height: 0},
shadowOpacity: 1,
borderWidth: Platform.OS === 'ios' ? 1 : 0,
shadowRadius: moderateScale(8),
elevation: moderateScale(8),
backgroundColor: Colors.white,
borderRadius: moderateScale(25),
overflow: 'hidden',
flexDirection: 'row',
}}>
<TextInput
placeholder={translate('search_parent')}
onChangeText={text => this.onChange(text)}
value={this.state.searchText}
style={{
borderWidth: 0,
color: 'black',
fontSize: moderateScale(16),
paddingLeft: moderateScale(20),
width: '85%',
fontFamily: Fonts.nunitoBold,
}}
/>
{this.state.searchText == '' ? (
<Image
source={Images.search_icon}
style={{
height: moderateScale(23),
width: moderateScale(23),
top: moderateScale(5),
margin: moderateScale(8),
}}
/>
) : (
<TouchableOpacity
onPress={() => {
this.clearSearchBox();
}}>
<Image
source={Images.close_pink}
style={{
height: moderateScale(18),
width: moderateScale(18),
top: moderateScale(5),
margin: moderateScale(8),
}}
/>
</TouchableOpacity>
)}
</View>
);
}
Step 3 – Search functionality
Here we are going to provide the definition of search function, which is performed on change of text.
onChange(searchText) {
this.setState({searchText: searchText});
if (searchText !== null && searchText !== undefined && searchText
!== '') {
const {parentData} = this.state;
var newArray = [];
newArray = parentData.filter(function(text) {
let user_name = text.first_name + ' ' + text.last_name;
return
user_name.toLowerCase().includes(searchText.toLowerCase());
});
newArray !== null && newArray !== undefined && newArray.length > 0
? this.setState({
parentData: newArray,
isSearch: true,
})
: console.log('==========', this.state.parentData);
} else {
this.getParentApiCall(false);
}
}
Step 4 – For clear search box
clearSearchBox() {
setTimeout(() => {
this.setState({searchText: '', isSearch: false});
}, 100);
this.getParentApiCall(false);
}
Step 4 – Complete code for search functionality
Here you find the whole code for searching data from a list of array object.
import React, {Component} from 'react';
import {
View,
SafeAreaView,
StyleSheet,
Image,
Text,
TextInput,
TouchableOpacity,
Alert,
TouchableWithoutFeedback,
} from 'react-native';
import {Colors, Images, Strings, Fonts} from '../themes';
import {Actions, ActionConst} from 'react-native-router-flux';
import NetInfo from '@react-native-community/netinfo';
const TAG = 'Parent';
export default class Parent extends Component {
constructor(props) {
super(props);
this.state = {
parentData: [], //storing API response
isSearch: false,
isAddParent: false,
searchText: '',
};
}
onChange(searchText) {
this.setState({searchText: searchText});
if (searchText !== null && searchText !== undefined && searchText !== '') {
const {parentData} = this.state;
var newArray = [];
newArray = parentData.filter(function(text) {
let user_name = text.first_name + ' ' + text.last_name;
return;
user_name.toLowerCase().includes(searchText.toLowerCase());
});
newArray !== null && newArray !== undefined && newArray.length > 0
? this.setState({
parentData: newArray,
isSearch: true,
})
: console.log('==========', this.state.parentData);
} else {
this.getParentApiCall(false);
}
}
clearSearchBox() {
setTimeout(() => {
this.setState({searchText: '', isSearch: false});
}, 100);
this.getParentApiCall(false);
}
render() {
return (
<View
style={{
margin: moderateScale(15),
marginRight: moderateScale(30),
width: '90%',
shadowColor: Colors.shadow,
shadowOffset: {width: 0, height: 0},
shadowOpacity: 1,
borderWidth: Platform.OS === 'ios' ? 1 : 0,
shadowRadius: moderateScale(8),
elevation: moderateScale(8),
backgroundColor: Colors.white,
borderRadius: moderateScale(25),
overflow: 'hidden',
flexDirection: 'row',
}}>
<TextInput
placeholder={translate('search_parent')}
onChangeText={text => this.onChange(text)}
value={this.state.searchText}
style={{
borderWidth: 0,
color: 'black',
fontSize: moderateScale(16),
paddingLeft: moderateScale(20),
width: '85%',
fontFamily: Fonts.nunitoBold,
}}
/>
{this.state.searchText == '' ? (
<Image
source={Images.search_icon}
style={{
height: moderateScale(23),
width: moderateScale(23),
top: moderateScale(5),
margin: moderateScale(8),
}}
/>
) : (
<TouchableOpacity
onPress={() => {
this.clearSearchBox();
}}>
<Image
source={Images.close_pink}
style={{
height: moderateScale(18),
width: moderateScale(18),
top: moderateScale(5),
margin: moderateScale(8),
}}
/>
</TouchableOpacity>
)}
</View>
);
}
}
So we completed the react native search functionality in this tutorial which is “How to implement search in react native“.
You can find my post on medium as well click here please follow me on medium as well.
You can find my next post here.
If have any query/issue, please feel free to ask.
Happy Coding Guys.
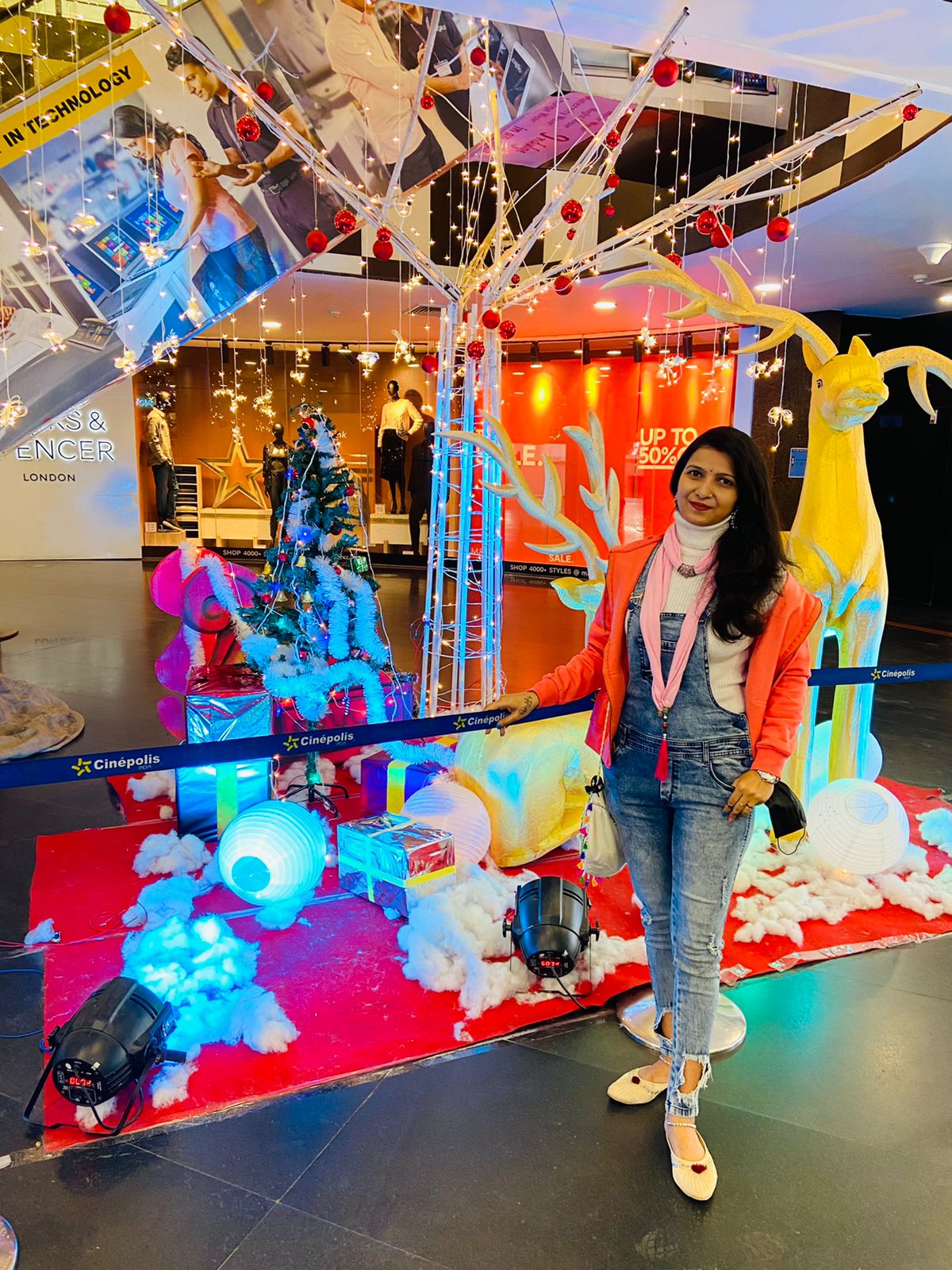
Hi, I am a professional Ionic and React Native Pixel Perfect App Designer and Developer, with expertise in Client Communication, Bug Fixing, Third Party Lib, Version Control Tools, Requirement Understanding, and managing teams, I have 6+ years of experience in the same domain as well as in Codeigniter, JS, IoT, and more than 10 other languages. For the last 6+ years, not a single day went without design/development.
Please follow me on Medium: https://nehadwivedi1004.medium.com/