Operation on image:
So let’s start the topic “Pick image from gallery and click image using camera in ionic“, using camera or gallery for picking image in any app is very important part of app.
Output Example –
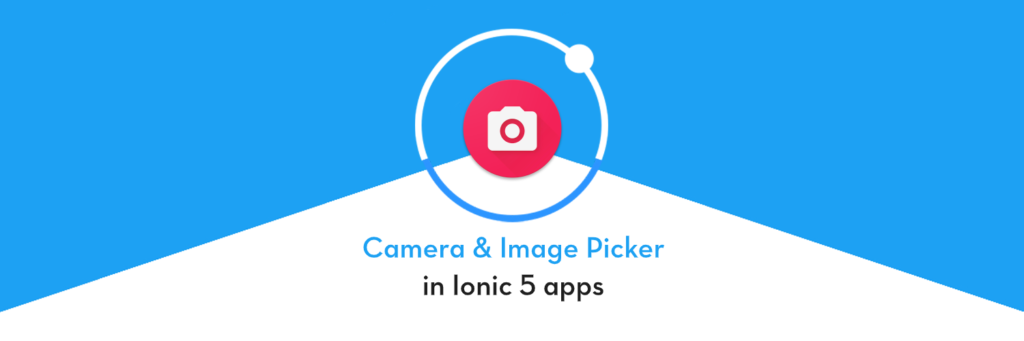
Follow these steps for picking the image from gallery and camera.
Step1:
Add and install both the plugins first.
ionic cordova plugin add cordova-plugin-camera
npm install –save @ionic-native/camera
ionic cordova plugin add cordova-plugin-telerik-imagepicker –variable PHOTO_LIBRARY_USAGE_DESCRIPTION=”your usage message”
npm install –save @ionic-native/image-picker
Step2:
Now we import these plugins in app.module.ts and also add in provider.
import { ImagePicker } from ‘@ionic-native/image-picker’;
import { Camera, CameraOptions } from ‘@ionic-native/camera’;
Now adding this in provider
providers: [
ImagePicker,
{provide:Camera]
})
Step3:
Now we ready to create design of page .html file
<ion-header>
<ion-navbar color=”primary”>
<ion-title align=”center”>Upload Image</ion-title>
</ion-navbar>
</ion-header>
<ion-content padding>
<ion-card>
<ion-card-header class=”color_blue”>
<b>Upload Image Using Camera and Gallery</b>
</ion-card-header>
<ion-card-content>
<ion-row>
<ion-col col-9>
<img src=”assets/imgs/camera.png” class=”fileupload col_mg_img” (click)=”takeSelfie()” />
</ion-col>
<ion-col col-6>
<img src=”assets/imgs/upload.png” class=”fileupload col_mg_img” (click)=”imagepicker()” />
</ion-col>
</ion-row>
</ion-card-content></ion-card>
</ion-content>
Step4:
Now we ready to implement logic in .ts file
import {NavController, NavParams, ToastController, Platform, Events} from ‘ionic-angular’;
import {Camera, CameraOptions} from “@ionic-native/camera”;
import {ImagePicker} from “@ionic-native/image-picker”;
@Component({
selector: ‘page-home’,
templateUrl: ‘home.html’
})
export class HomePage {
imagedata: any=[];
constructor(private imagePicker:ImagePicker, public camera:Camera, public navParams:NavParams) {}
takeSelfie():void {
this.camera.getPicture({
quality: 95,
destinationType: this.camera.DestinationType.DATA_URL,
sourceType: this.camera.PictureSourceType.CAMERA,
allowEdit: true,
encodingType: this.camera.EncodingType.PNG,
targetWidth: 500,
targetHeight: 500,
saveToPhotoAlbum: true
}).then(profilePicture => {
this.imagedata = profilePicture;
}, error => {
// Log an error to the console if something goes wrong.
console.log(“ERROR -> ” + JSON.stringify(error));
});
}
imagepicker() {
this.camera.getPicture({
quality: 95,
destinationType: this.camera.DestinationType.DATA_URL,
sourceType: this.camera.PictureSourceType.SAVEDPHOTOALBUM,
allowEdit: true,
encodingType: this.camera.EncodingType.PNG,
targetWidth: 500,
targetHeight: 500,
saveToPhotoAlbum: true
}).then(profilePicture => {
this.imagedata = profilePicture;
}, error => {
// Log an error to the console if something goes wrong.
console.log(“ERROR -> ” + JSON.stringify(error));
});
}
}
Why i choose ionic –
I’m a big fan of ionic with angular, because it is very easy to implement complicated concepts and designs, in above example i am explaining you in detail how to pick images from gallery and how to use camera in app.
if you have any query or doubt regarding this post or any of my post please comment, i’ll reply as soon as possible.
So we complete the tutorial which is “Pick image from gallery and click image using camera in ionic“.
You can find my post on medium as well click here please follow me on medium as well.
You can find my next post here.
If have any query/issue, please feel free to ask.
Happy Coding Guys.
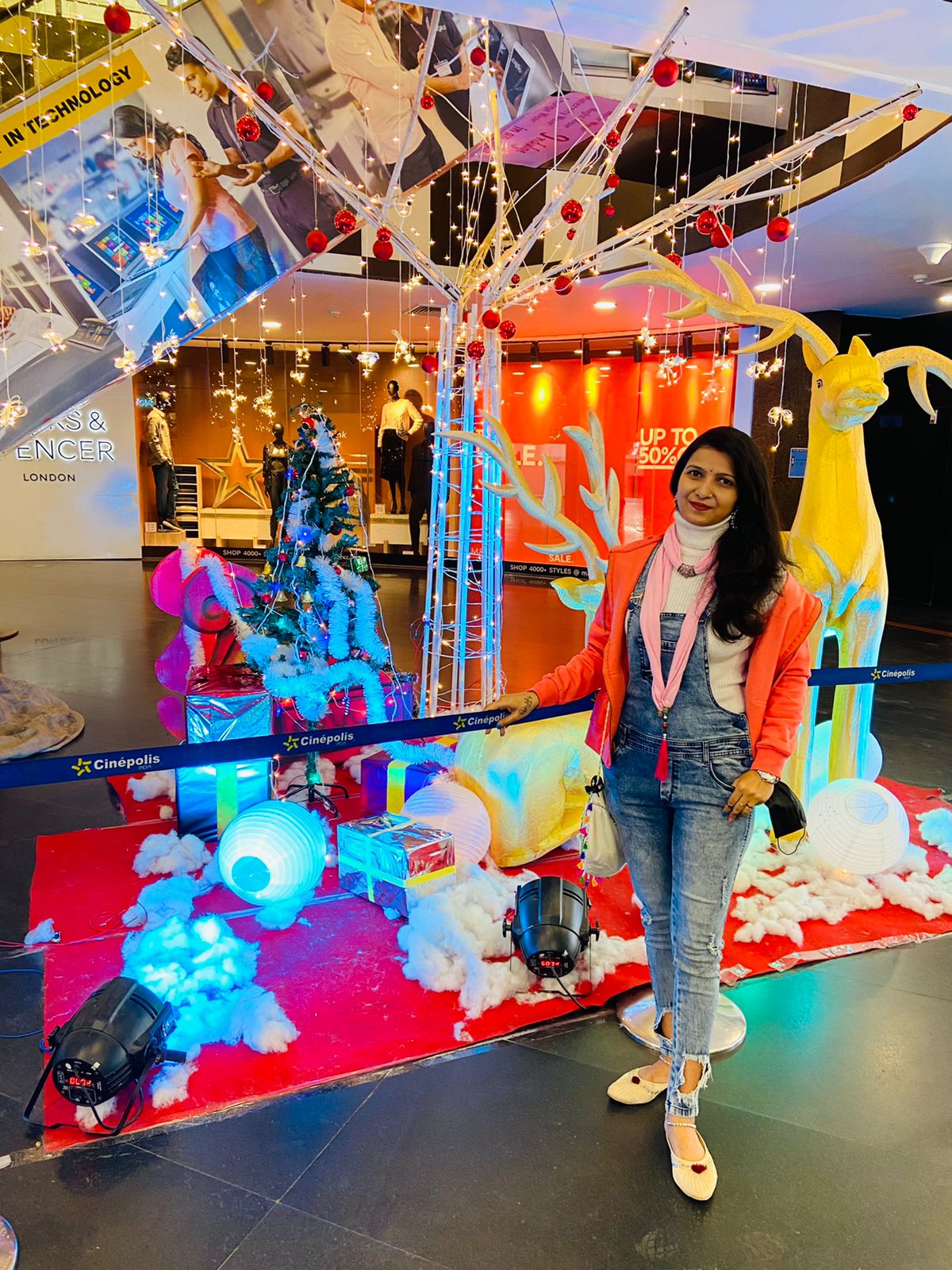